Usage
HornSchunck() class is written in Python.
It returns optical flow velocities (u,v) for given parameters:
- alpha : (float) the regularization coefficient of smoothness constraint
- maxIter : (int) maximum number of iterations required to obtain flow velocities
- applyGauss : (bool) apply Gaussian smoothing as a preprocess {False by default}
- GaussKernel : (tuple) Gaussian Filter kernel size {(15,15) by default}
optFlow = HornSchunck(alpha=alpha,maxIter=maxIter)Then use estimate() method to calculate optical flow velocities (u,v). After execution, getFlowField() method returns the colorized flow field.
optFlow.applyGauss = True
optFlow.GaussKernel = (5,5)
(u,v) = optFlow.estimate(frame1,frame2)Prediction of target frame from anchor via flow velocities is possible with predict() method. Moreover, getCollage() method puts 4 frames together to show a collage.
flowField = optFlow.getFlowField()
anchorP = optFlow.predict()
collage = optFlow.getCollage(f1_idx,f2_idx)
Implementation Details
- Gaussian smoothing process is added as an optional preprocessing and highly recommended to apply to obtain better visual saturation on the flow field.
- While calculating the image and temporal derivatives Ex, Ey, Et below filters are used as a 2D convolution operands.
- The flow field velocities u and v are initialized with all zero matrices with a same shape of first given frame.
- Another 3x3 filter is used for the estimation of the Laplacian of the flow velocities as proposed in the reference paper. [1]
- New set of velocity estimates are computed from the estimated derivatives and the average of the previous velocity estimates. Also, smoothing parameter (alpha) placed in the denominator of this iterative update rule.
- After observations on the iteration limit, one more condition is added such that if relative error starts to grow breaks the loop. Max iteration size is no longer an important parameter thanks to this condition.
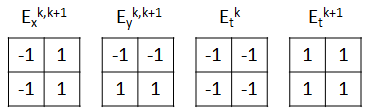
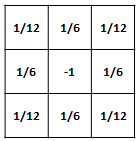

Reference Paper
[1] Horn and Schunck. (1981). Determining Optical Flow
Credits
Utilized from OpticalFlow_Visualization by Tom Runia (tomrunia@github) for optical flow field colorization.